Node.js Package
DeepScan provides a Node.js package which enables you to see bugs and quality issues in the CLI (command-line interface) or programmatically.
Overview
DeepScan for Node.js helps you to see bugs and quality issues in the CLI (command-line interface). As for the code review, you can check the code by this package on your CI.
- Analyze a whole project folder or specific folder/files.
- Report issues by format.
- Ignore rules or pattern of files.
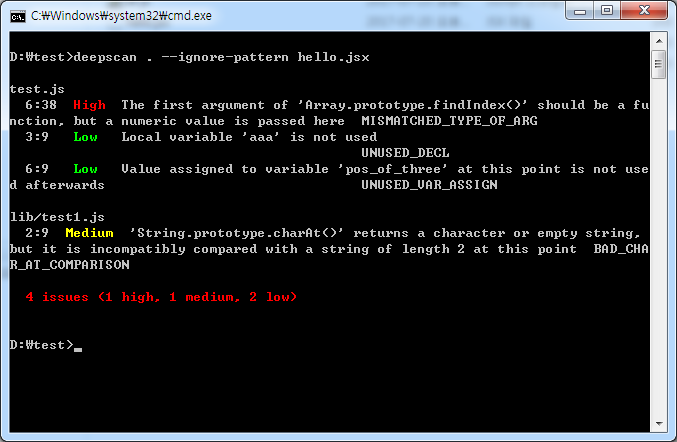
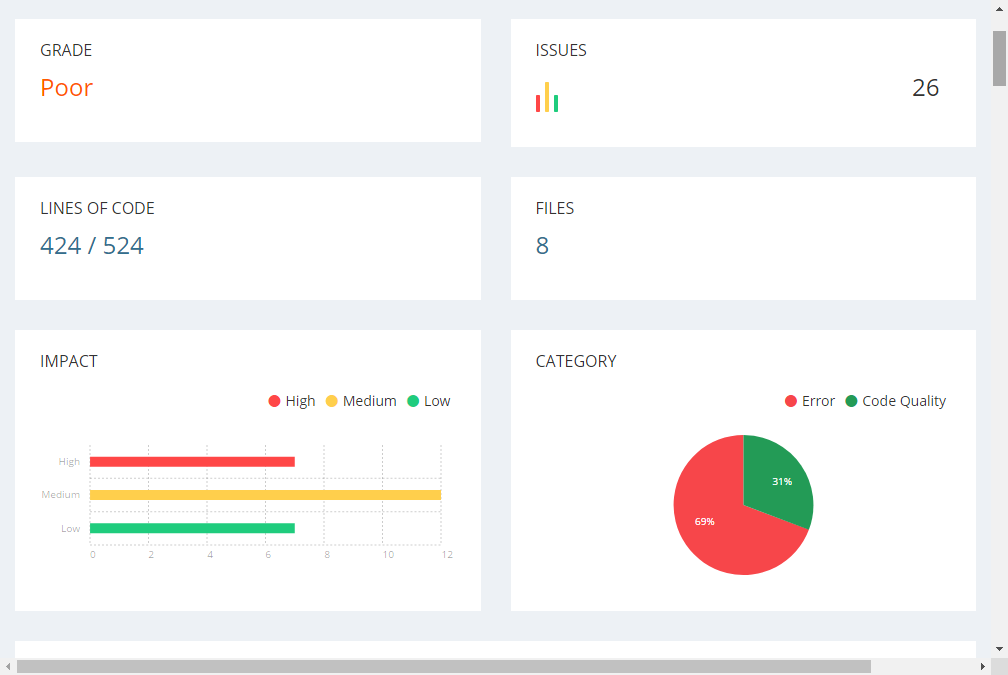
Requirements
To run this package, you need Java 8 (Oracle's JDK or OpenJDK).
Installation
There are two ways to install DeepScan: globally and locally.
Note:
This package uses java package to invoke Java-based analysis engine. When you have problems in the installation, please see here.
On Windows, I recommend to install windows-build-tools as an administrator, which easily installs all required tools.npm install --global --production windows-build-tools
Local Installation
If you want to include DeepScan as part of your project's build system, we recommend installing it locally.
$ npm install deepscan --save-dev
Or if you have a tarball:
$ npm install deepscan-<version>.tgz
After that, you can run DeepScan on any file or directory like this:
$ ./node_modules/.bin/deepscan --license <license_file_path> yourfile.js
Global Installation
If you want to make DeepScan available to tools that run across all of your projects, we recommend installing DeepScan globally.
$ npm install -g deepscan
Or if you have a tarball:
$ npm install -g deepscan-<version>.tgz
After that, you can run DeepScan on any file or directory like this:
$ deepscan --license <license_file_path> yourfile.js
Settings
DeepScan for Node.js provides the following options.
-l, --license
Specify DeepScan license file.
deepscan . -l <license_file_path>
-f, --format
Use a specific output format. (defaults to stylish
)
deepscan . -l <license_file_path> -f json
stylish
is a color-coded textcsv
is a CSV stringhtml
is a HTML string (a standalone report with analysis results and charts)json
is a JSON string
-o, --output-file
Specify file to write report to.
deepscan . -l <license_file_path> -f json -o ./result.txt
--no-color
Strip color codes from the output.
deepscan . -l <license_file_path> --no-color -o ./result.txt
--ignore-rules
Specify rules to ignore.
deepscan . -l <license_file_path> --ignore-rules "UNUSED_DECL,UNUSED_VAR_ASSIGN"
--ignore-patterns
Specify pattern of files to ignore. Each pattern follows the gitignore format.
deepscan . -l <license_file_path> --ignore-patterns "lib/,*.jsx"
--eslint-enable
Run ESLint. You can see the ESLint alarms with DeepScan's.
deepscan . -l <license_file_path> --eslint-enable
eslint package is required in the local or global. Note that NODE_PATH
environment variable is necessary to load the eslint
module installed in global.
It directly uses the package so your custom configurations and plugins are applied as is.
Below is an example of webpack build using DeepScan command-line tool with ESLint. Both DeepScan alarms (NULL_POINTER) and ESLint alarms (no-unused-vars, ...) are detected and you can control your build process by these alarms or an exit code.
--eslint-merge
Option for how identical issues of DeepScan and ESLint are merged. (defaults to deepscan
)
deepscan . -l <license_file_path> --eslint-enable --eslint-merge both
deepscan
: Show only DeepScan issueseslint
: Show only ESLint issuesboth
: Show all issues as is
Exit Code
0
when no issues are reported1
when only low-impact issues are reported2
when high or medium-impact issues are reported
Analysis
Analysis Target
For detailed information about the analysis target, refer to the following:
DeepScan API
It’s possible to use DeepScan programmatically through the Node.js API. You can use DeepScan functionality directly through the API.
var deepscan = require('deepscan');
// Initialize DeepScan with license
deepscan.init({ license: '<license_file_path>' });
var results = [];
// For file or directory
results = deepscan.verifyFile('/home/test/hello');
// For source text
results = deepscan.verify('var foo = null; foo.g;');
// For source text (TypeScript)
results = deepscan.verify('export class RuleActionProvider implements vscode.CodeActionProvider { var foo = null; foo.g; }', {
language: 'ts'
});
// For source text (TypeScript React)
results = deepscan.verify("abstract class Hello extends Component { render() { var foo = null; foo.g; return <RoleSelect />; }}", {
language: 'tsx'
});
const { alarms } = results;
for (const { impact, name, message, filePath, location, codeFragment } of alarms) {
console.log(`${name}:${message}`);
}